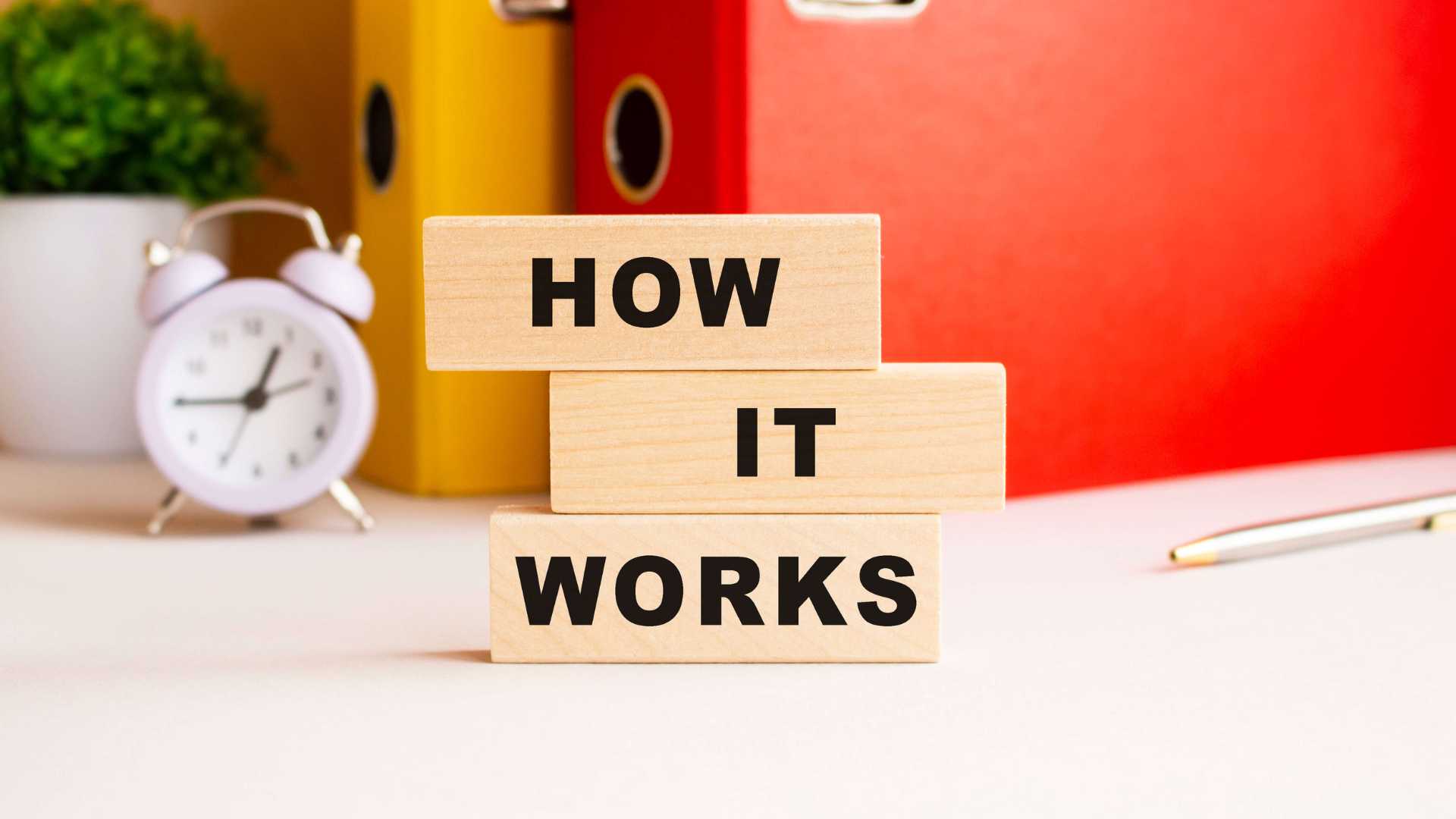
Selenium WebDriver: What It is, How It Works, and If You Need It
It’s perfectly reasonable for even experienced software developing teams to find themselves asking, “what is Selenium?” After all, the world of automated testing is still relatively new, even if it is incredibly important to the future of the software industry.
For starters, Selenium is a portable, open-source framework designed for the rapid testing of web applications. The Selenium web driver is just one part of the overall suite, which also contains the Selenium Remote Control, Selenium IDE, and Selenium Grid.
The program is unique in that it makes use of a test domain language called Selenese to write test scripts. All of the above tools have their own unique functions inside the Selenium suite, allowing programmers to assign different aspects of the program to different projects as needed.
How does the Selenium web driver work specifically? Well, it allows users to execute cross-browser tests to ensure that their new application is running as expected. This is a major time-saver, as it presents a simple, concise programming interface in one compact bundle.
What Is the Selenium WebDriver Used For?
To give you an impression of what Selenium can be used for, we’ll spend a bit more time talking about the scriptwriting process. For instance, thanks to its cross-platform interface, Selenium testers can write a script in one programming language and then re-use that same script across multiple browser programs. In fact, Selenium is compatible with a wide range of such programs, including:
Google Chrome
Internet Explorer 7-11
Firefox
Safari
Opera
Android
iOS
HtmlUnit
phantomJS
Again, the primary benefit of having something like Selenium in our corner is that it allows us to shorten the testing process via increased automation. This, in turn, reduces the time-to-market, time-to-delivery, and research and development time outlined above. In gaining control of these factors, we can drastically reduce the overall costs associated with software development.
Understanding the Advantages of Selenium WebDrivers
In this section, we’ll outline just some of the ways that Selenium has helped us take our client’s efforts to the next level.
Java can be used to execute Selenium commands, which means there is no need to configure a separate environment just for running Selenium
Selenium can be quickly and seamlessly integrated with Spring, which helps developers connect their web applications to a variety of data stores (including relational and non-relational databases, cloud-based data services, and map-reduce frameworks).
Selenium supports a wide array of frameworks, including Maven, Junit, and TestNG. This makes it easier for programmers to further automate the testing process. The suite also supports CI and CD tools like Jenkins, which can aid in automating the deployment process.
As we already mentioned, Selenium offers testers the ability to use a variety of different browsers, easily switching between them in seconds to ensure proper function.
Supports multiple operating systems, including Windows, Mac, Linux, Unix, etc.
Selenium is also able to mimic user input. For instance, in real scenarios, you can automate events such as key presses, drag and drops, mouse clicks, selecting, etc.
Selenium is also compatible with a wide range of languages, from .Net to Java to PHP, Python, and Ruby.
Using Selenium allows testers to greatly speed up the test process, especially when compared to other tools designed for the same purpose.
The suite is compatible with AndroidDriver, iPhoneDriver, and HtmlUnitDriver, allowing it to support web applications with both images and video.
Selenium offers high levels of visibility in the end-to-end testing of applications and programs.
The Selenium suite is also highly compatible with other tools, including Appium, Sikuli, ExtentReports, and more. This drastically improves flexibility and gives testers and developers a wider grip on the overall project. In the case of ExtentReports, testers can easily generate graphs and charts to give the entire team better insight into the status of the project.
Of course, most of these points apply mainly to programmers.
The primary reason for a business or organization to invest in Selenium programming is the suite’s high level of transparency, which ensures that developers, QAs, clients, and management can all stay on the same page. There is also the added benefit of platform independence, which saves countless tester man-hours by using the same test script across multiple platforms.
As we already stated, Selenium can drastically speed up both time-to-market and time-to-delivery, saving costs and preventing calls and emails from dissatisfied clients. By shortening and automating as much of the testing process as possible, companies can boost their productivity by leaps and bounds. In fact, the Selenium framework’s multi-platform functionality does not only reduce testing time but has also been shown to greatly improve testing quality.
How Selenium WebDriver Works
The Selenium WebDriver’s functionality is split into three steps:
The JSON wire protocol converts all test commands into an HTTP request.
Every browser has its own unique driver, which can be initialized on the server before any test cases are executed.
Once everything is in place, the browser can start receiving requests via the driver.
Below, you can see an example of a piece of code:
WebDriver driver = new ChromeDriver();
driver.get (https://https://inventorsoft.co/)
Once you have the code written, you can execute the program. Keeping with the example code, this will execute the Chrome browser, which will then navigate to the BrowserStack site. Now, between you clicking the “run” button and the launching of the browser, a couple of interesting things are taking place.
For example, upon execution, every line of code and script is transformed into a URL by the JSON wire protocol. Each URL is then passed over to the browser driver (in this case, the Chrome Driver). From here, the client library (which is Python in our example) translates the code into a JSON format, which looks like this:
https://localhost:8080/{"url":https://https://inventorsoft.co/"}
Of course, in order to receive the HTTP requests, every individual Browser Driver has to use an HTTP server. So once the driver is fed the URL, it can process the request by handing it over to the actual browser via HTTP. This allows for all the commands in your Selenium scripts to be executed automatically.
Configuring the WebDriver
Depending on your specific needs, there may be a variety of ways in which you want to configure your WebDriver. Some of the most commonly-used options include the following:
Headless – This ensures that the browser will be launched without a user interface.
No-Sandbox – This configuration disables Chrome’s sandbox, potentially restricting Selenium’s ability to affect browser processes.
Window-size=1280x800 – This configuration allows the tester to specify the window size of the browser.
Ignore-certificate-errors – This configuration tells the driver to ignore any expired or invalid TLS certificates, essentially bypassing those error pages.
Disable-dev-shm-usage – With this configuration, testers can disable the shared memory used by Docker and instead ensure those files are written to /tmp. If you’re running an application in Docker without this option, it will fail.
Configuring the Selenium WebDriver for Spring
As we mentioned above, Selenium can be quickly and rather seamlessly integrated with Spring. This can help developers connect their web apps to the data stores of their choice.
Set the system property with the WebDriver path value:
webdriver.chrome.driver for Chrome
webdriver.gecko.driver for Firefox.
@Bean
@Scope(value = ConfigurableBeanFactory.SCOPE_PROTOTYPE)
public WebDriver webDriver() {
WebDriver webDriver = getChromeDriver(); // getFirefoxDriver();
return webDriver;
}
Locator Types
Selenium supports a total of eight different locators. This help defines an address that identifies a specific web element within a webpage. They are as follows:
By ID (which is the preferred locator)
By class name
By tag name
By linkText
By partial linkText
By CSS selector
By XPATH
Wait Types
Waits are needed in order to prevent Selenium from throwing up exceptions on elements that are not fully loaded. This can be particularly helpful when responses are big, or latency is high. There are three primary types:
Implicit - Directs the WebDriver to wait for a certain measure of time before throwing an exception. Implicit wait stays in place for the entire browser session. It is set to 0 by default but can be changed in driver config.
Explicit - Directs the WebDriver to wait for a certain condition before proceeding with code execution. It can be applied to specific elements only
- Fluent - Looks for a web element repeatedly at regular intervals. It repeats until timeout happens or until the object is found. Polling frequency may be adjusted as needed.
How to Use Selenium Driver
To demonstrate how to use the Selenium driver, we’ve included one of our own cases to serve as an illustration. For reference, our case was built on the web automation class.
Here, we have a BI platform written in Java, which allows us to visualize different data from different data sources. It also allows us to perform a variety of different tasks with this data. For example, in this case, a user operating on a self-set schedule can receive a letter from the screenshots of the graph he wants, and also include some attachments in ppt or Excel format.
This is where Selenium comes in.
First, we log in. If we were to reopen a session without authentication, nothing would work. To accomplish this, we use the “Find element” method. Depending on which element we specify, it returns either a single web element or a list of elements from which we can select the one we need.
Then, on the login page, we look for input with the types “email” and “password,” then send the credentials. This is an example of a chart that will be loaded after authentication is completed
After we’ve entered the login and password, we emulate clicking on the login button, then wait a few seconds for the entire page to load. Keep in mind that all the conditions we discuss may vary depending on your setup and configuration. Now, we have a logo or avatar as the condition for loading the page. Next, we follow the link. In our case, the link leads to a specific chart.
Once at this location, we need to wait a few more seconds for all the data to be pulled up and for the graph to be drawn. We now have the same element that contains the graph, so we can call up a screenshot of the image by setting “getScreenshotAs ()” on the web element type object.
From here, Selenium provides three main export options
BASE 64 - long row (String)
Byte array
To File
We can also take other screenshots in the same browser session
(Example of a Screenshot)
In the next example, we’ll be using nested charts from third-party services. These are developed on separate resources and then inserted into the Iframe. To begin, we open it like we would any other page, then log in to the third-party service (in our case, it is Tableau). After that, we will have full access to this element.
Now, how do we make the transition to iframe? Well, by default, Selenium can only work with the mainstream window, as each window has its own handle. We can get the handle by taking the web driver type's object and using the getWindowHandle method on it. Iframe also has its own separate handle. We can find the Iframe element on this page, and go to it through an object of the web driver type, then switch to() method and select the ID of the required frame.
Sample code to switch to an iframe:
WebElement iframe = webDriver.findElement(By.tagName("iframe"));
webDriver.switchTo().frame(iframe);
Here, we are already in the middle of the Iframe, which has its own HTML elements inside. Also, it’s important to note that the third-party service may require authentication. For example, we had a button for login, so we look for a button and click on it to log in. When we do so, a popup appears. Next, we need to go to the third handle - from iframe to a popup.
After opening the window, we select the window handle that is already there. From here, we open the last value that was made available to us. This will be a login form, and we simply enter the credits and then click on the Login button. After that, the popup closes, and we again need to pass to handle Iframe in order to work with it. Once authorized, the schedule (or, in this case, a map) will be loaded and we can work with it as usual.
In order to get access to graphics (maps), we need to be in the Iframe Handler and not in the main window. That said, if we have combinations with ordinary charts (which are either inserted through a JavaScript in DIVS directly into the main window or into Iframes) they will also work with the main handle. However, we will need to take a parent element for all graphics and work with them.
We can then save this as a file.
Next, let's take an example from different graphs and tables. Specifically, let’s take a common container in which all these graphs are placed.
Conclusion
The sheer competitiveness of the software development industry is putting a lot of stress on developers and their parent companies to cut time-to-market, time-to-delivery, and costs in general.
The primary way in which this can be accomplished without an increase in man hours (and therefore expenses) is through automation.
The Selenium suite’s high levels of transparency, compatibility, and platform independence are specifically designed to save time, save money, and boost tester productivity. Moreover, they are essential to ensuring that developers can deliver for their clients and avoid the delays that plague the industry, and prevent maximum profitability.
Related articles
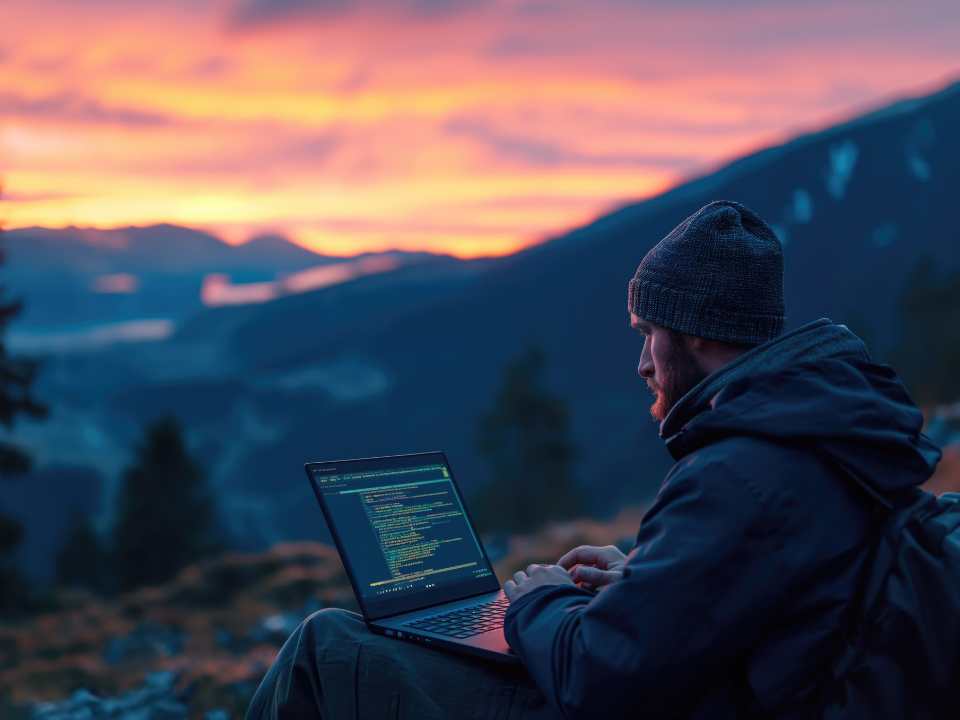
Easier and Faster Coding: How GitHub Copilot Brings Actual Benefits in Real Projects
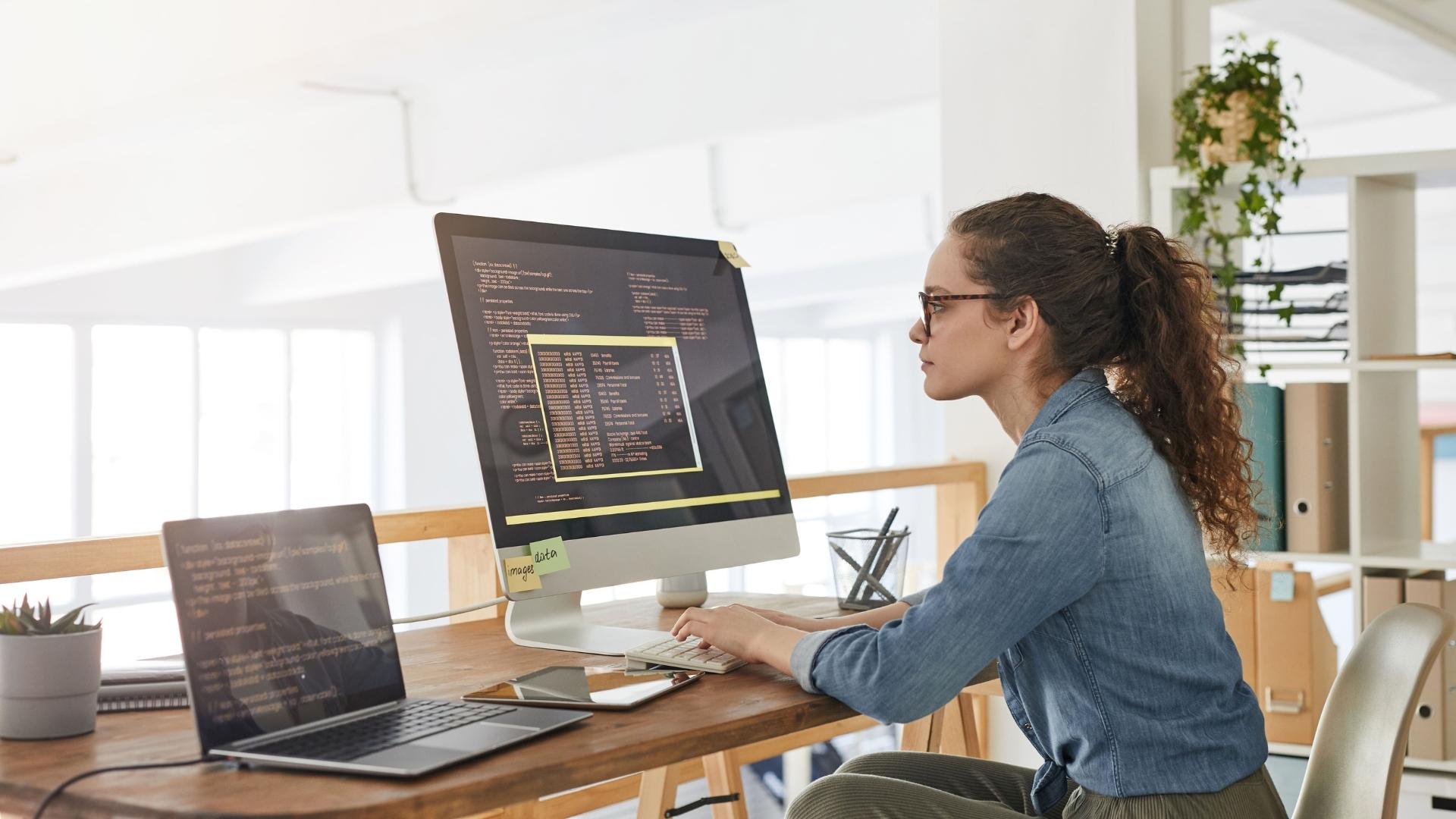
Top 10 Advantages of Microservices
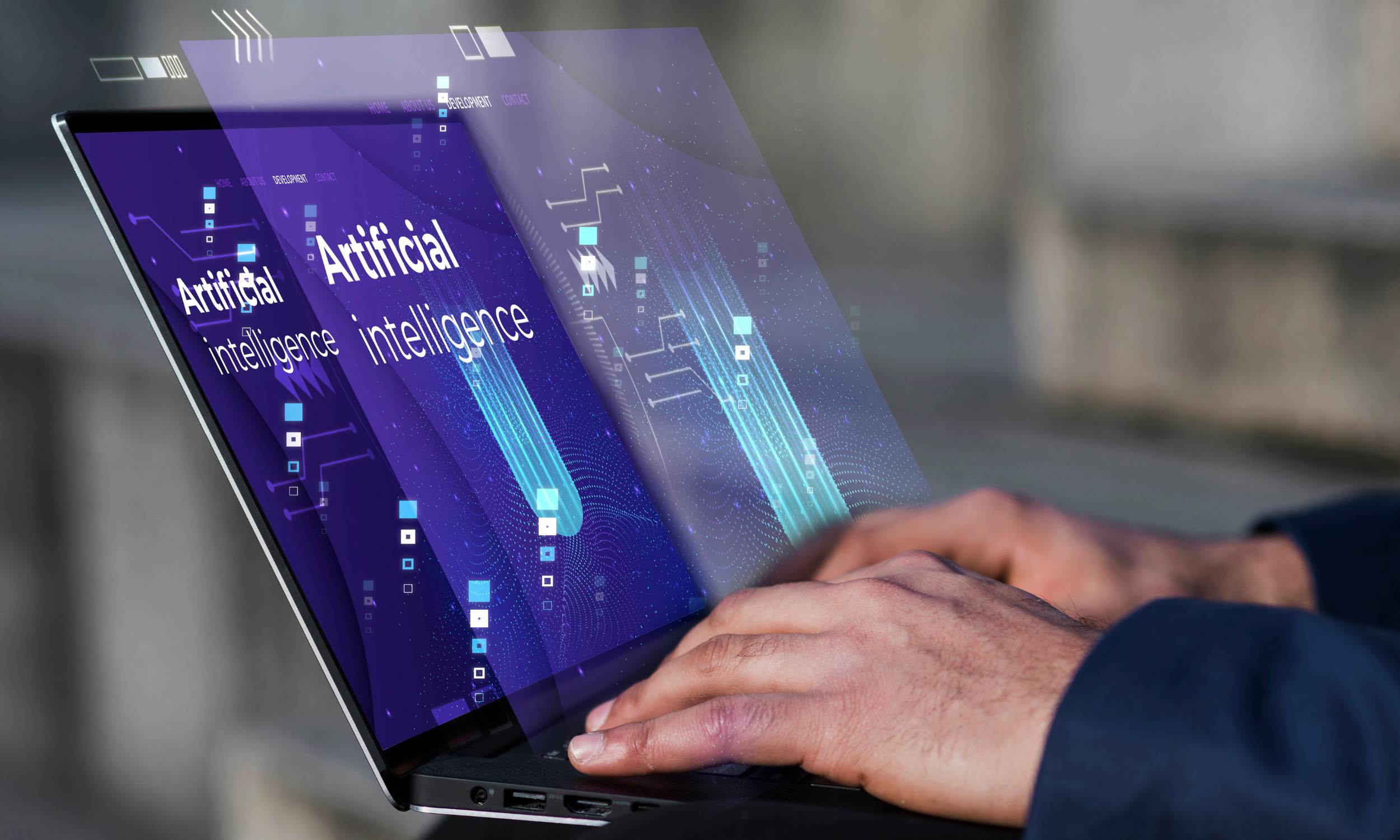
AI in SaaS - Top Ideas and Trends in 2024 that will make a difference
- What Is the Selenium WebDriver Used For?
- Understanding the Advantages of Selenium WebDrivers
- How Selenium WebDriver Works
- Configuring the WebDriver
- How to Use Selenium Driver
- Conclusion